Introduction
When working with dataframes in Pandas, it’s often necessary to remove the index column to clean up the data or prepare it for certain analyses. Whether you’re new to Pandas or a seasoned user, this comprehensive guide will provide a step-by-step explanation on how to remove the index column effortlessly.
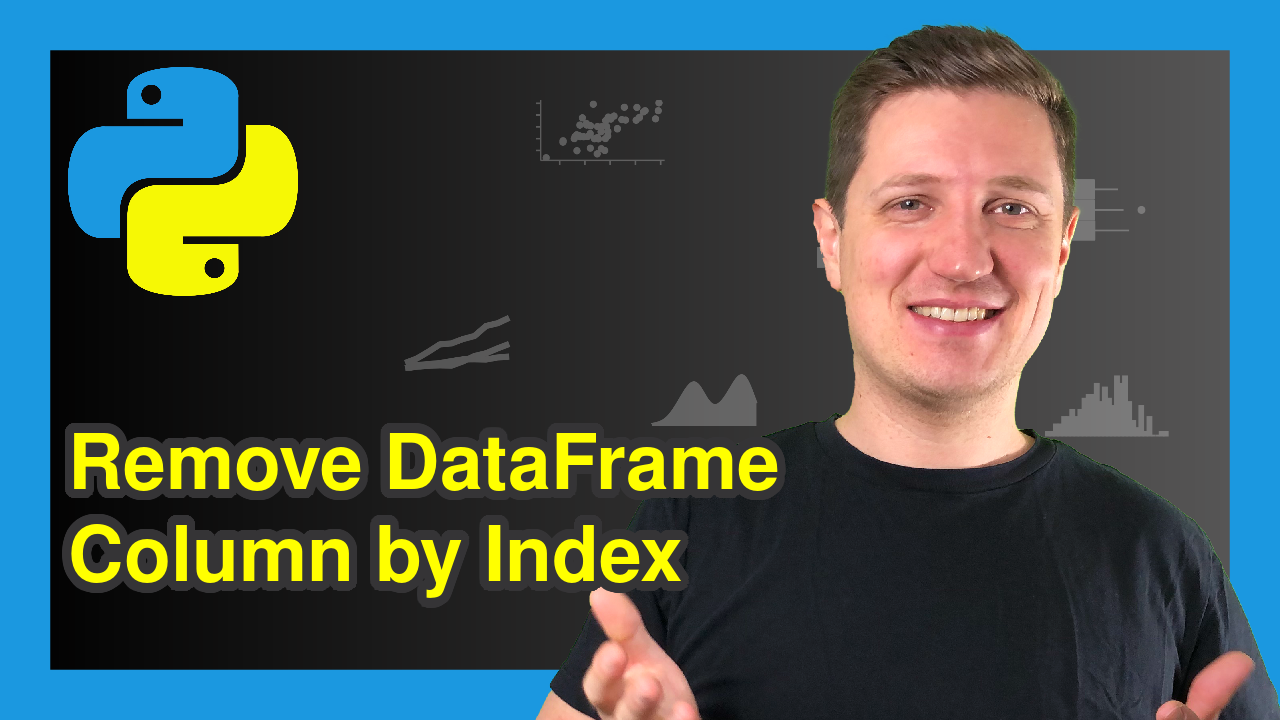
Image: statisticsglobe.com
Ready to dive in and learn the art of index column removal in Pandas? Let’s get started!
Removing the Index Column
To remove the index column in Pandas, you can utilize the .reset_index() method. This method takes one optional argument, **inplace**, which defaults to False. When inplace is set to True, the changes are made directly to the original dataframe. If it’s set to False, a new dataframe is returned without the index column.
Here’s the syntax:
df = df.reset_index(inplace=False)
Example:
import pandas as pdCreate a dataframe with an index
df = pd.DataFrame('Name': ['Alice', 'Bob', 'Carol'], 'Age': [20, 25, 30])
Remove the index column
df = df.reset_index(inplace=False)
Print the dataframe without the index
print(df)
Output:
Name Age 0 Alice 20 1 Bob 25 2 Carol 30
Alternative Method: Using the .drop() Method
Another way to remove the index column is to use the .drop() method. This method can be targeted to specifically remove the index using the following syntax:
df = df.drop('index', axis=1)
Example:
import pandas as pdCreate a dataframe with an index
df = pd.DataFrame('Name': ['Alice', 'Bob', 'Carol'], 'Age': [20, 25, 30])
Remove the index column using .drop()
df = df.drop('index', axis=1)
Print the dataframe without the index
print(df)
Output:
Name Age 0 Alice 20 1 Bob 25 2 Carol 30
FAQs on Index Column Removal
Q: Why should I remove the index column?
A: Removing the index column in Pandas is useful when you want to work with the data as a standard NumPy array or when you need to append or merge the dataframe with other data sources.
Q: What happens if I use the inplace=True argument?
A: If you set inplace to True, the changes will be made directly to the original dataframe, overwriting the existing data. This can be useful to avoid creating unnecessary copies, but it’s important to use with caution to prevent unintended data loss.
Q: When is it better to use the .drop() method instead of .reset_index()?
A: The .drop() method is more efficient when you only want to remove the index column and not reset the index labels. It’s a more direct approach for index column removal.
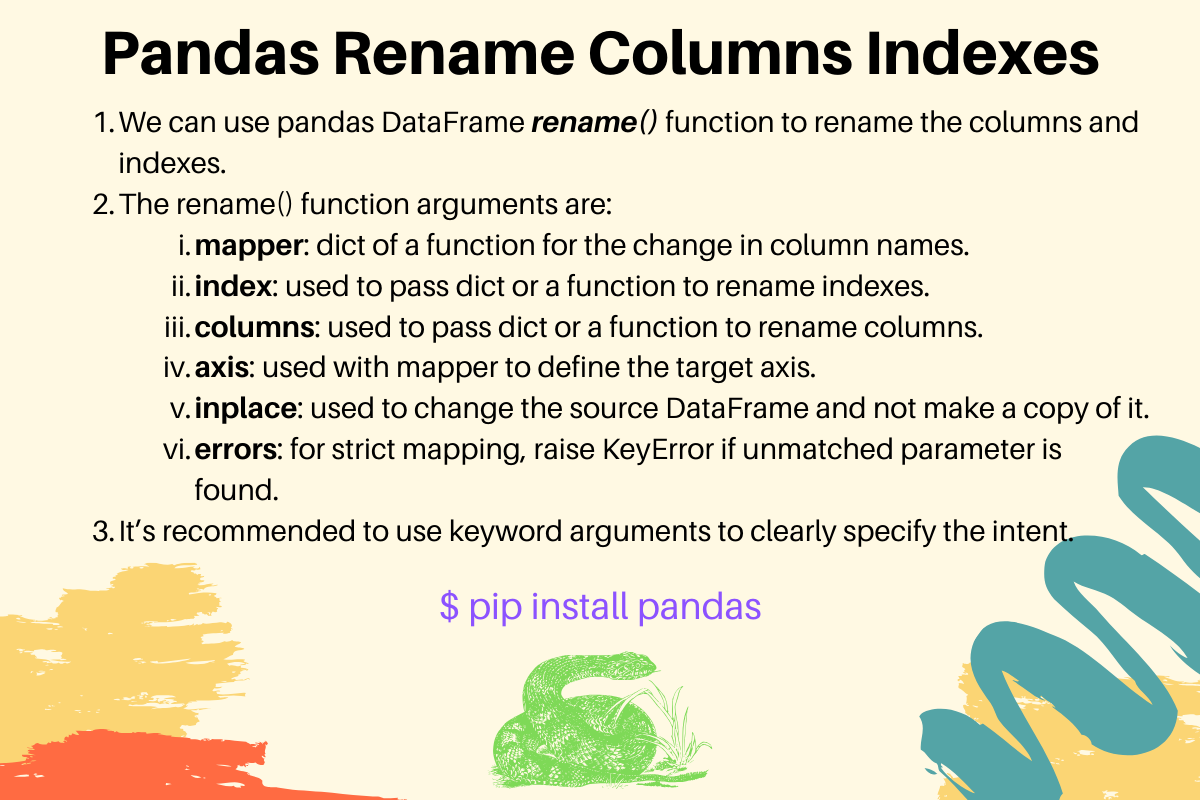
Image: www.digitalocean.com
How To Remove Index Column In Pandas
Conclusion
掌握了移除 Pandas 中的索引列的技巧,您現在可以輕鬆地清理數據並使其適合您的分析需求。無論您是處理大型數據集還是只是為小項目進行數據準備,這些知識都將使您的工作流程更加順暢。
如果您還有任何疑問,請不要猶豫,在下方留言。我們將很樂意提供幫助,讓您的 Pandas 知識再上一層樓!您是否對 Pandas 中的其他主題感興趣?如果您這樣做,請告訴我們,我們將很樂意創建更多有助於您擴展知識的文章。